Josh Marinacci has a blog entry on how to integrate Java FX into Swing applications: http://blogs.sun.com/javafx/entry/how_to_use_javafx_in.
It's good to have a final answer on how to accomplish this in JavaFX 1.0, but to be honest it looks like a nasty hack, which implies it's something Sun either hadn't thought about or didn't consider important. That seems peculiar as it was so easy to do in previous releases, and it seems a dumb move not to make Swing and JavaFX as easily interoperable as possible.
Saturday, December 20, 2008
Saturday, December 13, 2008
JavaFX and Swing - again
I've noticed that my previous entry about using JavaFX widgets in Swing applications has been getting a lot of traffic since JavaFX 1.0 was finally released.
Having finally had time to download and try out the JavaFX 1.0 SDK it seems like Sun have done a good job: the demos and samples are all good, JavaFX.com no longer looks embarrassing and the NetBeans integration is great (there is an Eclipse plugin available too).
However, the 1.0 release has removed the javafx.ext.swing.Canvas class, which was crucial in embedding JavaFX widgets in Swing applications, as the Canvas had a method to return a JComponent, so you could simply add it to your Swing application without too much trouble. Now I can't see any way of easily using JavaFX in Swing applications - I have heard some hints that it can be done with some reflection 'hacks', but I haven't seen any more information about this.
I hope there is a relatively simple way of achieving this, because if JavaFX really is 'Swing 2' then they need to make the two interoperate as seamlessly as possible so that people can migrate their existing Swing applications in small steps, rather than having to rewrite in JavaFX.
Having finally had time to download and try out the JavaFX 1.0 SDK it seems like Sun have done a good job: the demos and samples are all good, JavaFX.com no longer looks embarrassing and the NetBeans integration is great (there is an Eclipse plugin available too).
However, the 1.0 release has removed the javafx.ext.swing.Canvas class, which was crucial in embedding JavaFX widgets in Swing applications, as the Canvas had a method to return a JComponent, so you could simply add it to your Swing application without too much trouble. Now I can't see any way of easily using JavaFX in Swing applications - I have heard some hints that it can be done with some reflection 'hacks', but I haven't seen any more information about this.
I hope there is a relatively simple way of achieving this, because if JavaFX really is 'Swing 2' then they need to make the two interoperate as seamlessly as possible so that people can migrate their existing Swing applications in small steps, rather than having to rewrite in JavaFX.
Thursday, November 20, 2008
GPS and MapView in Android
Having spent an evening hacking around writing an Android application that uses the GPS position together with the MapView component to show both your current location latitude/longitude and visually I spent a lot of time sifting through sites and blogs that used out of date APIs and overlooked crucial steps, so I thought I'd document how to enable GPS access and MapView usage using the Android Eclipse plugin and the emulator. This will then work when running on an Android device.
Step 1: GPS Access
Basic GPS access is relatively straightforward. You can use code like this in your Activity.onCreate() to get the current position and register a listener for position updates:
<uses-permission android:name="android.permission.ACCESS_LOCATION/">
<uses-permission android:name="android.permission.ACCESS_GPS"/>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
<uses-permission android:name="android.permission.ACCESS_MOCK_LOCATION"/>
For running your application in the Android emulator you will need mock position data, as there is no live GPS data from the emulator.
The easiest way to do this is to launch the emulator from Eclipse and then switch to the DDMS perspective (Window -> Open Perspective -> Other). Your Eclipse should then look something like the picture on the right.
This shows you a device browser on the left, w ith an Emulator Control tab underneath. Scroll down the Emulator Control tab until you see the Location Controls section. This allows you to enter mock location data to use when running the Emulator - the best way is to use a KML file, which you can create from Google Earth, or download a default location file from http://www.sunlightlabs.com/earmarks/house_defense_earmarks_08.kml. Once this has been imported click the green play button in the Location Controls (KML) section and your application will receive location updates based on the contents of the KML file.
Step 2: Google MapView component
The Google MapView component allows you to embed the Google map component in your application, so we can combine our live location data with a visual display of that location on a map. Embedding the MapView component has a few "tricky" steps:
Add the MapView component to your Activity:
Once you have this, then to enable the MapView component you need to:
Step 1: GPS Access
Basic GPS access is relatively straightforward. You can use code like this in your Activity.onCreate() to get the current position and register a listener for position updates:
In addition to this you need to add permissions to your Android manifest file to allow your application access to the location services:
LocationManager lm = (LocationManager)getSystemService(Context.LOCATION_SERVICE);
List pro = lm.getAllProviders();
Location l = lm.getLastKnownLocation(LOCATION_SERVICE);
updatePositionOnScreen(l);
Intent i = new Intent(LOCATION_CHANGED);
LocationListener ll = new LocationListener() {
public void onLocationChanged(Location location) {
updatePositionOnScreen(location);
}
public void onProviderDisabled(String provider) {
}
public void onProviderEnabled(String provider) {
}
public void onStatusChanged(String provider, int status, Bundle extras) {
}
};
lm.requestLocationUpdates(LocationManager.GPS_PROVIDER, 30000, 100, ll);
<uses-permission android:name="android.permission.ACCESS_LOCATION/">
<uses-permission android:name="android.permission.ACCESS_GPS"/>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
<uses-permission android:name="android.permission.ACCESS_MOCK_LOCATION"/>
For running your application in the Android emulator you will need mock position data, as there is no live GPS data from the emulator.

The easiest way to do this is to launch the emulator from Eclipse and then switch to the DDMS perspective (Window -> Open Perspective -> Other). Your Eclipse should then look something like the picture on the right.
This shows you a device browser on the left, w ith an Emulator Control tab underneath. Scroll down the Emulator Control tab until you see the Location Controls section. This allows you to enter mock location data to use when running the Emulator - the best way is to use a KML file, which you can create from Google Earth, or download a default location file from http://www.sunlightlabs.com/earmarks/house_defense_earmarks_08.kml. Once this has been imported click the green play button in the Location Controls (KML) section and your application will receive location updates based on the contents of the KML file.
Step 2: Google MapView component
The Google MapView component allows you to embed the Google map component in your application, so we can combine our live location data with a visual display of that location on a map. Embedding the MapView component has a few "tricky" steps:
Add the MapView component to your Activity:
<com.google.android.maps.MapViewThe apiKey attribute should be the MD5 fingerprint of your Maps API key. In order to deploy your MapView enabled application you need to register for a Google Maps API key, but for debugging you can use the Android SDK's debug key. All the details on Google Maps API keys can be found here http://code.google.com/android/toolbox/apis/mapkey.html, with details on how to obtain the debug SDK fingerprint here http://code.google.com/android/toolbox /apis/mapkey.html#getdebugfingerprint.
android:id="@+id/mapview1"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:enabled="true"
android:clickable="true"
android:apiKey="your api key"
/>
Once you have this, then to enable the MapView component you need to:
- Change your Activity to extend MapActivity rather than Activity.
- Add <uses-library android:name="com.google.android.maps"/> as a child of the
element in the Android Manifest file. - Enable Internet access for your application so that it can download map data by adding <uses-permission android:name="android.permission.INTERNET"/> to your manifest file.
MapView mapView = (MapView) findViewById(R.id.mapview1);When you run your application you should see your live MapView control responding to GPS lcoation updates, like below:
MapController mc = mapView.getController();
GeoPoint p = new GeoPoint((int) (l.getLatitude() * 1E6),
(int) (l.getLongitude() * 1E6));
mc.animateTo(p);
mc.setZoom(16);
mapView.invalidate();

Thursday, November 13, 2008
Tracking Java versions of web site visitors
Gili over at 'Where the cows roam free" has a very interesting post about how to combine Google Analytics and the new Java deployment file to track what version of Java visitors to a website are using:
http://cowwoc.blogspot.com/2008/11/tracking-java-versions-using-google.html
It's trivial to add it to an existing Google Analytics-enabled site, and should hopefully yield some interesting and useful results, so I've added it to this site and Cool Applets too.
http://cowwoc.blogspot.com/2008/11/tracking-java-versions-using-google.html
It's trivial to add it to an existing Google Analytics-enabled site, and should hopefully yield some interesting and useful results, so I've added it to this site and Cool Applets too.
Labels:
java version
Monday, October 20, 2008
Java 6 Update 10 released
Looks like Java 6 Update 10 has been officially released - for some reason Sun seem to be keeping it quiet, which seems a little odd, considering how significant this release should be for client-side Java and Java FX.
Windows and Linux users go get it: http://java.sun.com/javase/downloads/index.jsp. OS X users, bang your head on your desk in frustration at Apple's lousy Java support.
Windows and Linux users go get it: http://java.sun.com/javase/downloads/index.jsp. OS X users, bang your head on your desk in frustration at Apple's lousy Java support.
Wednesday, October 08, 2008
Fail blog
A photo I took is potentially going to be included on Fail Blog - it's obviously the best one, so please vote it up!

more fail, owned and pwned pics and videos

more fail, owned and pwned pics and videos
Labels:
fail blog,
photography
Debugging Applets on OS X
So, despite Apple's best efforts to hide the Java Preferences dialog in OS X (Applications/Utilities/Java/Java Preferences in case you're still looking) enough people must have found it by now for Apple to move to the next step: cripple the dialog itself.
After installing the latest Java Update from Apple I noticed a redesigned Java Preferences dialog, which was certainly more compact than the previous version, mainly because Apple have removed the Applet runtime parameters field. So, if you want to set up your applet for remote debugging, change the default heap size or pass any other runtime parameter you now have to go an edit a file manually, as described at http://support.apple.com/kb/HT3210
This may be fine for developers, although I don't see how it is an improvement over editing via the dialog that was there before this update, but instructing non-technical users on how to set up debugging or heap parameters for applets just got more difficult for no good reason.
After installing the latest Java Update from Apple I noticed a redesigned Java Preferences dialog, which was certainly more compact than the previous version, mainly because Apple have removed the Applet runtime parameters field. So, if you want to set up your applet for remote debugging, change the default heap size or pass any other runtime parameter you now have to go an edit a file manually, as described at http://support.apple.com/kb/HT3210
This may be fine for developers, although I don't see how it is an improvement over editing via the dialog that was there before this update, but instructing non-technical users on how to set up debugging or heap parameters for applets just got more difficult for no good reason.
Sunday, September 07, 2008
Google Chrome and Java Applets
Well, loathe as I am to add yet another blog post to the insane amount of Chrome related items that were blogged and reported last week, I did notice something interesting with regard to Chrome running Java applets.
Pretty much the first thing I did after installing Chrome was to try it out with an applet as I wasn't sure whether Chrome supported applets or not. It did, it all seemed to work and I didn't think much more about it. It was only a day or two later after seeing some Java.net forum posts that I realised that Chrome only works with the latest version of Java SE, version 6 Update 10, which contains all the cool new features to make Java applets run better.
This could be a good thing - one thing I've said before is that Update 10 has some great features in it, but getting everyone to upgrade to it is going to be hard work. A lot of people, and especially most large corporations, do not regularly upgrade the version of Java SE on their desktop. So, if Chrome forces people to install the very latest version then great.
However, does Chrome explicitly tell users that they need that version of Java? Does it direct them to the right place to get it? If not, then it's just going to create more client-side Java confusion, and I've seen reports of Chrome not running Java properly even when Update 10 is present, and so it's great in principle to make people install the latest and greatest JRE, but unless it's a seamless and trouble-free experience to do so then it will not help Java's already tarnished client-side reputation.
Pretty much the first thing I did after installing Chrome was to try it out with an applet as I wasn't sure whether Chrome supported applets or not. It did, it all seemed to work and I didn't think much more about it. It was only a day or two later after seeing some Java.net forum posts that I realised that Chrome only works with the latest version of Java SE, version 6 Update 10, which contains all the cool new features to make Java applets run better.
This could be a good thing - one thing I've said before is that Update 10 has some great features in it, but getting everyone to upgrade to it is going to be hard work. A lot of people, and especially most large corporations, do not regularly upgrade the version of Java SE on their desktop. So, if Chrome forces people to install the very latest version then great.
However, does Chrome explicitly tell users that they need that version of Java? Does it direct them to the right place to get it? If not, then it's just going to create more client-side Java confusion, and I've seen reports of Chrome not running Java properly even when Update 10 is present, and so it's great in principle to make people install the latest and greatest JRE, but unless it's a seamless and trouble-free experience to do so then it will not help Java's already tarnished client-side reputation.
Sunday, August 31, 2008
Compiled JavaFX in Swing applications - again
Update: This doesn't work in the full release of JavaFX. Please see here for how to do this in Java FX 1.0.
I posted a while ago about problems I was having integrating a compiled JavaFX widget into a Swing application - theoretically it should have been possible, as it's all Java and Swing, but I hit a brick wall that looked like it was due to JavaFX being in early, early alpha/beta stage, so I left it and decided to come back to it when JavaFX was more stable.
Well, the preview SDK for JavaFX is now available, and I had a wet Sunday afternoon to spare, so I tried it again. Of course, as it has been 4 or 5 weeks since I last looked at JavaFX they have completely changed the structure and location of the classes, so it took me a while to get the hang of things again, but once I had done that actually getting a JavaFX widget into a Swing application was trivial really.

The screenshot above shows the Stopwatch example from the JavaFX SDK inside a Swing window - the two controls to the left are Swing controls.
The key to getting JavaFX to operate with Swing is the javafx.ext.swing.Canvas object, which has a getJComponent() method, so all you need to do is place all of your JavaFX content onto a Canvas object, then use the JComponent returned by Canvas.getJComponent() to add the JavaFX widget to any Swing container.
For the example above, the Stopwatch widget example runs in a JavaFX frame with no Canvas, but all we need to do is write a simple JavaFX Canvas object to wrap the Stopwatch, using the code below:
Once we have compiled that, we can use our JavaFX 'SWCanvas' object in our Swing application just like any other Java class, so the simple JFrame example pictured above does just that:
And that's all there is to it. The trickiest part of the whole thing was getting the classpath for the JavaFX runtime right when running the Swing application (your CLASSPATH should look something like: %JAVAFX_HOME%\lib\javafxgui.jar;%JAVAFX_HOME%\lib\javafxrt.jar;%JAVAFX_HOME%\lib\javafx-swing.jar;%JAVAFX_HOME%\lib\Scenario.jar).
With JavaFX's ability to create impressive animated user interfaces quickly, and with relatively little code, then the ability to add JavaFX widgets to any Swing container could be a great way to improve Swing applications incrementally, rather than rewriting the whole application in JavaFX.
I'm definitely not a JavaFX expert though, so if you want some more ideas of the very impressive things that you can do with JavaFX then check out James Weaver's JavaFX Blog.
I posted a while ago about problems I was having integrating a compiled JavaFX widget into a Swing application - theoretically it should have been possible, as it's all Java and Swing, but I hit a brick wall that looked like it was due to JavaFX being in early, early alpha/beta stage, so I left it and decided to come back to it when JavaFX was more stable.
Well, the preview SDK for JavaFX is now available, and I had a wet Sunday afternoon to spare, so I tried it again. Of course, as it has been 4 or 5 weeks since I last looked at JavaFX they have completely changed the structure and location of the classes, so it took me a while to get the hang of things again, but once I had done that actually getting a JavaFX widget into a Swing application was trivial really.

The screenshot above shows the Stopwatch example from the JavaFX SDK inside a Swing window - the two controls to the left are Swing controls.
The key to getting JavaFX to operate with Swing is the javafx.ext.swing.Canvas object, which has a getJComponent() method, so all you need to do is place all of your JavaFX content onto a Canvas object, then use the JComponent returned by Canvas.getJComponent() to add the JavaFX widget to any Swing container.
For the example above, the Stopwatch widget example runs in a JavaFX frame with no Canvas, but all we need to do is write a simple JavaFX Canvas object to wrap the Stopwatch, using the code below:
package stopwatch;
import javafx.ext.swing.Canvas;
import javax.swing.JComponent;
public class SWCanvas {
public function getChildComponent() {
var sw = StopwatchWidget{}
var c2:Canvas = Canvas {
content: sw
width: 400
height: 400
visible: true
}
return c2.getJComponent();
}
}
Once we have compiled that, we can use our JavaFX 'SWCanvas' object in our Swing application just like any other Java class, so the simple JFrame example pictured above does just that:
package stopwatch;
import javax.swing.*;
import java.awt.Dimension;
import java.awt.FlowLayout;
public class SwingStopwatch {
public static void main(String[] args) {
Runnable r = new Runnable() {
public void run() {
new SwingStopwatch().runMe();
}
};
SwingUtilities.invokeLater(r);
}
public void runMe() {
JFrame jf = new JFrame("Swing and JavaFX Test");
jf.setPreferredSize(new Dimension(600,400));
jf.getContentPane().setLayout(new FlowLayout());
jf.getContentPane().add(new JButton("Click me"));
jf.getContentPane().add(new JTextField("Type into me"));
// JavaFX widget here!
stopwatch.SWCanvas c1 = new stopwatch.SWCanvas();
jf.getContentPane().add(c1.getChildComponent());
jf.pack();
jf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jf.setVisible(true);
}
}
And that's all there is to it. The trickiest part of the whole thing was getting the classpath for the JavaFX runtime right when running the Swing application (your CLASSPATH should look something like: %JAVAFX_HOME%\lib\javafxgui.jar;%JAVAFX_HOME%\lib\javafxrt.jar;%JAVAFX_HOME%\lib\javafx-swing.jar;%JAVAFX_HOME%\lib\Scenario.jar).
With JavaFX's ability to create impressive animated user interfaces quickly, and with relatively little code, then the ability to add JavaFX widgets to any Swing container could be a great way to improve Swing applications incrementally, rather than rewriting the whole application in JavaFX.
I'm definitely not a JavaFX expert though, so if you want some more ideas of the very impressive things that you can do with JavaFX then check out James Weaver's JavaFX Blog.
Friday, August 01, 2008
JApplet and the Swing EDT
So, everyone knows by now that doing anything relating to Swing controls when you're not running in the Swing Event Dispatch Thread (EDT) is a big no-no. Unfortunately this is very easy to do, as Swing doesn't enforce this rule and 90% of the time your code will run with no visible problems if you don't do it (right up until you release it to a customer, at which point bugs will become immediately visible).
A lot of the time it's reasonable that Swing doesn't force execution onto the EDT, certainly in high-level application classes, although the closer you get to the Swing classes the more sense it makes to actually enforce the rule in some way.
For instance when writing an application it's up to the developer to get on the Swing EDT before you create and display your Swing controls. However there are other situations in which it's obvious that Swing controls are going to be used and so Swing should put you on the EDT by default: when you're using JApplet for example.
I hadn't noticed before now but I was surprised to notice that JApplet.init() is not invoked on the EDT. Try it:
I guess the issue with doing it by default is that people who don't know about the EDT rule wouldn't be aware that it is being done for them, so you're just avoiding potential bugs without people learning what is involved in avoiding the bugs, so they may just get into another EDT problem further down the line. That may be true, and Swing is definitely an API not a 'framework', allowing you to write code without any help or guidance on how things should be done correctly along the way, but I still think that you should at least start people off on the right track whenever you can - what they do after that is up to them.
A lot of the time it's reasonable that Swing doesn't force execution onto the EDT, certainly in high-level application classes, although the closer you get to the Swing classes the more sense it makes to actually enforce the rule in some way.
For instance when writing an application it's up to the developer to get on the Swing EDT before you create and display your Swing controls. However there are other situations in which it's obvious that Swing controls are going to be used and so Swing should put you on the EDT by default: when you're using JApplet for example.
I hadn't noticed before now but I was surprised to notice that JApplet.init() is not invoked on the EDT. Try it:
Now if you're using a JApplet you obviously want to do Swing stuff in your applet, so why not help people out and start them off on the Swing EDT?
public class TestApplet extends JApplet {
public void init() {
System.out.println("JApplet.init - EDT = " +
SwingUtilities.isEventDispatchThread());
}
}
I guess the issue with doing it by default is that people who don't know about the EDT rule wouldn't be aware that it is being done for them, so you're just avoiding potential bugs without people learning what is involved in avoiding the bugs, so they may just get into another EDT problem further down the line. That may be true, and Swing is definitely an API not a 'framework', allowing you to write code without any help or guidance on how things should be done correctly along the way, but I still think that you should at least start people off on the right track whenever you can - what they do after that is up to them.
Tuesday, July 15, 2008
JavaScript and Groovy service functions in AltioLive
As discussed in the Using AltioLive with JavaScript whitepaper (http://developers.altio.com/developer/library/JavaScriptwithAltio.pdf), Java 6.0 has built in support for dynamic scripting languages, such as JavaScript and Groovy, within the JVM (referred to as JSR 223).
That document discussed integrating that functionality with the AltioLive client as a custom control, but you can also utilize the same feature to add support for implementing AltioLive Service Functions with scripting languages. We'll take a quick look at how to implement this in Java 6.0 and earlier JVMs by using the Apache Bean Scripting Framework (BSF).
All that is required is a simple Java service function proxy to handle calling a script and returning the results. There are 2 service functions, one for Java 6 and one for BSF, and the source code is included with the Altio script service function JAR file (http://developers.altio.com/developer/downloads/scriptsvcfunc.jar). Each service function takes the same parameters:
The advantages to using a scripting language for custom Altio Service Functions instead of Java are:
Java6
3 - Install the Altio Script Service Function by copying the JAR file (http://developers.altio.com/developer/downloads/scriptsvcfunc.jar) into (Altio\WEB-INF\lib)
4 - Create an Altio\WEB-INF\classes\scripts folder and copy the example scripts (http://developers.altio.com/developer/downloads/scripts.zip) in there.

Example JavaScript:
Results:
Execute Groovy Service Function

Example Groovy:
Results:
These are trivial examples, but they demonstrate how you can implement Altio Service Functions in dynamic scripting languages. Their advantages of ease of development and deployment should make it simple to start using them for more complicated tasks. Further enhancements to the proxy could include passing parameters to the named script's service function, which would make it possible to send values all the way from the AltioLive client through to a server side script function.
That document discussed integrating that functionality with the AltioLive client as a custom control, but you can also utilize the same feature to add support for implementing AltioLive Service Functions with scripting languages. We'll take a quick look at how to implement this in Java 6.0 and earlier JVMs by using the Apache Bean Scripting Framework (BSF).
All that is required is a simple Java service function proxy to handle calling a script and returning the results. There are 2 service functions, one for Java 6 and one for BSF, and the source code is included with the Altio script service function JAR file (http://developers.altio.com/developer/downloads/scriptsvcfunc.jar). Each service function takes the same parameters:
- Engine: Name of the script engine to use. e.g. javascript, js, groovy
- Script: Name of the script to execute
- Function: Name of the function in the script to execute
The advantages to using a scripting language for custom Altio Service Functions instead of Java are:
- Quicker development time (no IDE or compiler required)
- Take advantage of particular langauge features to perform tasks. Some languages are better at string handling, XML parsing etc and are better suited for particular tasks than Java.
Setup:
1 - Either:- Run the AltioLive Presentation Server using a Java 6 JDK and use the Java 6 examples.(You should remove 'eics-client.jar' from Altio\WEB-INF\lib before starting otherwise you will have JSP compilation problems)
- Run AltioLive Presentation Server using an older JDK and use the BSF examples
Java6
- There are lots of engines here: https://scripting.dev.java.net/files/documents/4957/37593/jsr223-engines.zip
- For each engine you'll need supporting libraries.
- e.g. Groovy libraries can be found here: http://dist.groovy.codehaus.org/distributions/groovy-binary-1.5.6.zip
- Altio installs BSF by default, but I had to upgrade to 2.4.0 to get this to work.
- To add JavaScript support use Rhino: http://www.mozilla.org/rhino/download.html (copy the js.jar into WEB-INF\lib)
- Other engine can be found here: http://jakarta.apache.org/bsf/index.html
3 - Install the Altio Script Service Function by copying the JAR file (http://developers.altio.com/developer/downloads/scriptsvcfunc.jar) into (Altio\WEB-INF\lib)
4 - Create an Altio\WEB-INF\classes\scripts folder and copy the example scripts (http://developers.altio.com/developer/downloads/scripts.zip) in there.
Running
1 - You can reuse the Java Scripting service function proxy to execute any function in any script in any supported scripting engine, as in the examples below. The screenshots below are for the Java 6 implementation - if you are using the BSF then replace the classname withcom.altio.services.scripting.BSFScriptServiceFunctionExecute JavaScript Service Function

Example JavaScript:
function returnSomeXml() {
var result = "<javascript msg="'hello">"
return result;
}
Results:
<RESULT AL_ID="RESULT">
<javascript msg="hello again from javascript, the time is Tue Jul 15 2008 12:17:02 GMT+0100 (BST)" AL_ID="RESULT-javascript"/>
</RESULT>
Execute Groovy Service Function

Example Groovy:
def returnSomeXml2() {
String result = "<groovy>"
xml = {result+="<groovyitem id='${it}'/>"}
val = (1..10).collect(xml)
result += "</groovy>"
return result
}
Results:
<RESULT AL_ID="RESULT">
<groovy AL_ID="RESULT-groovy">
<groovyitem id="1" AL_ID="gi1"/>
<groovyitem id="2" AL_ID="gi2"/>
<groovyitem id="3" AL_ID="gi3"/>
<groovyitem id="4" AL_ID="gi4"/>
<groovyitem id="5" AL_ID="gi5"/>
<groovyitem id="6" AL_ID="gi6"/>
<groovyitem id="7" AL_ID="gi7"/>
<groovyitem id="8" AL_ID="gi8"/>
<groovyitem id="9" AL_ID="gi9"/>
<groovyitem id="10" AL_ID="gi10"/>
</groovy>
</RESULT>
These are trivial examples, but they demonstrate how you can implement Altio Service Functions in dynamic scripting languages. Their advantages of ease of development and deployment should make it simple to start using them for more complicated tasks. Further enhancements to the proxy could include passing parameters to the named script's service function, which would make it possible to send values all the way from the AltioLive client through to a server side script function.
Labels:
altio,
altiolive,
groovy,
javascript,
scripting
Friday, July 04, 2008
Swurl
Yet another social web aggregator site, to compete with FriendFeed and Socialthing, but Swurl does present the aggregated information in a really cool timeline format, which for me gives it the edge over the others, even though it's lacking generic RSS support at the moment.

Try it out: http://peakyblinder.swurl.com/timeline

Try it out: http://peakyblinder.swurl.com/timeline
Labels:
swurl
AltioLive and Applets at JavaOne
As I mentioned at the time I was interviewed on video about AltioLive and Applets at the Altio booth at JavaOne. If you can stand to see a slightly 'relaxed' man talk about Applets for 10 minutes then watch the video below!
Friday, June 27, 2008
Hudson CI game - again

We've fixed the build and upgraded to the fixed version. I'm keeping the points though, they're mine!
Wednesday, June 25, 2008
Using Java 6 Update 10 features in AltioLive
I've just posted an item on the Altio developer's blog about using the new features of Java 6 Update 10 in AltioLive applications. You can read the whole thing here:
http://tinyurl.com/42ntx2
The only issue at the moment is the fact that Altio dynamically constructs the APPLET tag, so we need to use a JSP page to generate a JNLP file dynamically. You can simply update the Altio JavaScript file and add in the new JNLP generator to start using cool new features in your AltioLive applications such as bigger heap sizes and separate JVMs per applet.
One thing that I didn't mention on the Altio blog is I that played around with accessing other domains directly from the applet, which I blogged about here.
I implemented this in Altio by allowing Image controls to fetch images from Flickr directly, rather then needing a Service Function to act as a proxy, and this worked well. It is probably a feature more useful in consumer internet applications rather than enterprise applications, but not being able to reference images from other websites directly has always been slightly frustrating, so it was a good thing to get working.
http://tinyurl.com/42ntx2
The only issue at the moment is the fact that Altio dynamically constructs the APPLET tag, so we need to use a JSP page to generate a JNLP file dynamically. You can simply update the Altio JavaScript file and add in the new JNLP generator to start using cool new features in your AltioLive applications such as bigger heap sizes and separate JVMs per applet.
One thing that I didn't mention on the Altio blog is I that played around with accessing other domains directly from the applet, which I blogged about here.
I implemented this in Altio by allowing Image controls to fetch images from Flickr directly, rather then needing a Service Function to act as a proxy, and this worked well. It is probably a feature more useful in consumer internet applications rather than enterprise applications, but not being able to reference images from other websites directly has always been slightly frustrating, so it was a good thing to get working.
Monday, June 23, 2008
Cross-domain unsigned applets
Wow, I completely missed this in the Java 6 Update 10 release notes: it is now possible for unsigned Java applets to connect to servers other than the one it was served from, which means you can easily implement client-side mashups with an applet without having to sign it.
http://weblogs.java.net/blog/joshy/archive/2008/05/java_doodle_cro.html
The great thing about this is that it doesn't really break the sandbox security model, which still protects the user's local machine from any damage from malicious applet code, but it makes applets a whole lot more useful in the Web 2.0 world.
http://weblogs.java.net/blog/joshy/archive/2008/05/java_doodle_cro.html
The great thing about this is that it doesn't really break the sandbox security model, which still protects the user's local machine from any damage from malicious applet code, but it makes applets a whole lot more useful in the Web 2.0 world.
Tuesday, June 17, 2008
Rebranding
I've had the marketing people in, and they have assessed the synergy of the blog with a view to maximising my ability to incentivize visionary channels. Therefore, out goes 'disagreeable surprises' (which was a pain in the arse to keep typing) and in comes Peaky Blinder.
For those interested, it's the name of a gang that were infamous in Birmingham in the early 20th century. The story is that a lot of my Dad's side of the family were members, and they had a reputation as being pretty nasty. Officially the name comes from them wearing caps low over their eyes, but according to family stories it's actually related to the fact that they kept razor blades in the peaks of their caps, so when a fight kicked off they used their caps as weapons to try and slash their opponents' eyes. Yow!
So, that's my new brand ;-)
For those interested, it's the name of a gang that were infamous in Birmingham in the early 20th century. The story is that a lot of my Dad's side of the family were members, and they had a reputation as being pretty nasty. Officially the name comes from them wearing caps low over their eyes, but according to family stories it's actually related to the fact that they kept razor blades in the peaks of their caps, so when a fight kicked off they used their caps as weapons to try and slash their opponents' eyes. Yow!
So, that's my new brand ;-)
Hudson CI game - FAIL
We lost our CVS server for a while today, so Hudson jobs started failing because they couldn't check out the source. Fair enough. However, my attention was drawn to the line 'This build was worth 12730.0 points" line for the failed build, so when I looked at the details I saw this:
So, a failed build awards thousands of points for removing all of our checkstyle and findbug errors, and subtracts 10 points for breaking the build? Not exactly the behaviour I was expecting, and some people I know might see that as an incentive to check in and break the build :-) And what happens when you fix the build - does it subtract thousands of points for suddenly adding a load of errors?

Wednesday, June 11, 2008
Downsizing
One of our raffle prizes at JavaOne was a 4GB Asus Eee pc/umpc/laptop thing. We had a play around with it before we reluctantly gave it away, and it is quite a cool little toy - very small and light, but with a half-decent keyboard and wi-fi. The only bad points were the screen (very small) and the need to boot the thing into 'advanced mode' and perform some black-hat magic to get a Java plugin installed for Firefox. It really wasn't trivial, and was certainly beyond the scope of the couple of hours we had to play around with it.
So, obviously when I was back in the UK and discovered there was a new Asus Eee out with more storage and a bigger screen, then I had to go and have a look, and when I played with one in the shop and discovered that it had a Java plugin installed and ran the Altio website demos without any problems then I was sold.

The screenshot above is the AltioLive applet running in Firefox on the Asus.
Now, I bought the Linux version of the Asus Eee PC900, but I'm going to have to crap all over my geek credentials by admitting that I installed XP on it after a couple of days. The version of Linux on the Eee seemed fine, but a bit noddy and didn't support multi-user logins easily, so I decided to switch to XP.
There followed a long, long, long time playing around with nLite, which slipstreams Windows installations by allowing you to decide what components to keep and what to remove. After a lot of trial and error, messing about with booting off USB drives (which was made easier by helpful pages such as http://www.eeeguides.com/2007/11/installing-windows-xp-from-usb-thumb.html), and finally giving up and buying an external USB CD drive, I finally got it installed.
As long as you are fairly brutal with what is installed in XP, and keep an eye on the services and startup programs you have, it runs really well on the Eee, even with its wheezy processor. With XP, I get to use the new Java 6 Update 10 plugin on the Eee too, so I may even see if I can use it for development, although I think trying to run Eclipse on it might be stretching a point too far.
So, obviously when I was back in the UK and discovered there was a new Asus Eee out with more storage and a bigger screen, then I had to go and have a look, and when I played with one in the shop and discovered that it had a Java plugin installed and ran the Altio website demos without any problems then I was sold.

The screenshot above is the AltioLive applet running in Firefox on the Asus.
Now, I bought the Linux version of the Asus Eee PC900, but I'm going to have to crap all over my geek credentials by admitting that I installed XP on it after a couple of days. The version of Linux on the Eee seemed fine, but a bit noddy and didn't support multi-user logins easily, so I decided to switch to XP.
There followed a long, long, long time playing around with nLite, which slipstreams Windows installations by allowing you to decide what components to keep and what to remove. After a lot of trial and error, messing about with booting off USB drives (which was made easier by helpful pages such as http://www.eeeguides.com/2007/11/installing-windows-xp-from-usb-thumb.html), and finally giving up and buying an external USB CD drive, I finally got it installed.
As long as you are fairly brutal with what is installed in XP, and keep an eye on the services and startup programs you have, it runs really well on the Eee, even with its wheezy processor. With XP, I get to use the new Java 6 Update 10 plugin on the Eee too, so I may even see if I can use it for development, although I think trying to run Eclipse on it might be stretching a point too far.
Thursday, June 05, 2008
Applet to applet comunication
Of course, after a talk demonstrating Applet to JavaScriipt and Applet to Flash communication the first question I had after my JavaOne session completely stumped me: how can 2 Applets on the same page communicate with each other?
Here's the solution - use the getAppletContext() method on your Applet (or JApplet) to get the context (page) of your applet and then find all the other Applets on the same page:
However, one of the new features in Java 6 Update 10 is to be able to specify that your Applet gets its own instance of the JVM. You can either do this explicitly with the following Applet parameter:
If your applet gets its own JVM, then it will not see other Applets on the same page because they are not in its context any more - there is no longer a single global JVM context for the page, which is correct from a technical point of view, but will mean that there doesn't seem to be a reliable way to enable Applet to Applet communication without using something like JavaScript as a communication bridge between them.
Here's the solution - use the getAppletContext() method on your Applet (or JApplet) to get the context (page) of your applet and then find all the other Applets on the same page:
Enumeration e = this.getAppletContext().getApplets();Once you have a reference to an Applet instance then you can call any public method on it that you need to.
while (e.hasMoreElements()) {
Applet a = (Applet)e.nextElement();
String name = a.getName();
System.out.println("Found applet " + name);
}
However, one of the new features in Java 6 Update 10 is to be able to specify that your Applet gets its own instance of the JVM. You can either do this explicitly with the following Applet parameter:
<param name="separate_jvm" value="true">or your applet will get one by default if it asks for a non-standard heap size, or JNLP support.
If your applet gets its own JVM, then it will not see other Applets on the same page because they are not in its context any more - there is no longer a single global JVM context for the page, which is correct from a technical point of view, but will mean that there doesn't seem to be a reliable way to enable Applet to Applet communication without using something like JavaScript as a communication bridge between them.
Sunday, May 25, 2008
Are applets making a comeback?
An interesting article over at JavaWorld dicusses that very topic:
http://www.javaworld.com/javaworld/jw-05-2008/jw-05-applets.html
http://www.javaworld.com/javaworld/jw-05-2008/jw-05-applets.html
Thursday, May 22, 2008
JavaOne Session Examples
The code examples from the JavaOne Technical Session that I presented a few weeks ago are now available from the Altio site, so for the people who attended the session and asked for the code, head over to http://www.altio.com/DeveloperCentre/Developers/JavaOne2008-Session-Examples.aspx and download all the Java source and HTML files that I used.
I haven't included the Altio application examples, but I can make them available as well if anyone needs them.
I haven't included the Altio application examples, but I can make them available as well if anyone needs them.
Tuesday, May 20, 2008
Compiled JavaFX in Swing applications
[Update: I got this working with the JavaFX preview release - see this blog entry]
I've integrating interpreted JavaFX script widgets into Swing applications by using the Java 6 Scripting Engine to execute the script and have the JavaFX widget add its Canvas component to a passed in Swing parent component to allow it to integrate with the Swing application seamlessly.
So, after returning from JavaOne I was keen to integrate compiled JavaFX widgets in a similar way, to give better performance and easier deployment of multi-script JavaFX code. I thought it would be fairly simple, but I hit a few hurdles along the way.
My first idea was to extend a custom Java class in JavaFX to easily add the widget to Swing. I hit a snag when I couldn't figure out how to implement constructors in JavaFX that Java can recognise.
I tried things like:
So I gave up on that and just defined an interface in Java that I then implemented in JavaFX, and that went well for a while - it's easy enough to implement interfaces in JavaFX, and I could obtain a reference to the JavaFX implementation from Java and call methods on it. So, if I call a method on the JavaFX widget that returns a JComponent then I can add that as a child of a Swing container and have integration that way, right?
Well, maybe, but I seem to have a fundamental JavaFX problem that's stopping it working. The same code works when I run the JavaFX script from the command line:
I'm presuming this is because the JavaFX SDK is not finalised, that I'm using the latest OpenJFX compiler in a beta version of the Java6 JVM and I've finally tipped over the bleeding edge onto the other side where nothing works. But still, it should work, I think, so if anyone has any ideas please let me know.
I've integrating interpreted JavaFX script widgets into Swing applications by using the Java 6 Scripting Engine to execute the script and have the JavaFX widget add its Canvas component to a passed in Swing parent component to allow it to integrate with the Swing application seamlessly.
So, after returning from JavaOne I was keen to integrate compiled JavaFX widgets in a similar way, to give better performance and easier deployment of multi-script JavaFX code. I thought it would be fairly simple, but I hit a few hurdles along the way.
My first idea was to extend a custom Java class in JavaFX to easily add the widget to Swing. I hit a snag when I couldn't figure out how to implement constructors in JavaFX that Java can recognise.
I tried things like:
public class javafxtest {
public function javafxtest(val1: String; val2 : String) : javafxtest {
return this;
}
}
But whatever I did in JavaFX, Java only saw an empty constructor and another that took a boolean.So I gave up on that and just defined an interface in Java that I then implemented in JavaFX, and that went well for a while - it's easy enough to implement interfaces in JavaFX, and I could obtain a reference to the JavaFX implementation from Java and call methods on it. So, if I call a method on the JavaFX widget that returns a JComponent then I can add that as a child of a Swing container and have integration that way, right?
Well, maybe, but I seem to have a fundamental JavaFX problem that's stopping it working. The same code works when I run the JavaFX script from the command line:
At runtime in my Swing application I get the following Exception when I call that JavaFX method:
public function setupCanvas() {
var c3:Canvas = Canvas {
content :
Text {
content: "A JavaFX widget!"
}
}
}
And, er, that's as far as I can get.
Caused by: java.lang.NoSuchMethodError: javafx.ui.Canvas.(Z)V
at javafxtest.altiotest.setupCanvas$impl(altiotest.fx:77)
at javafxtest.altiotest.setupCanvas(altiotest.fx:28)
at javafxtest.altiotest.getControlComponent$impl(altiotest.fx:101)
I'm presuming this is because the JavaFX SDK is not finalised, that I'm using the latest OpenJFX compiler in a beta version of the Java6 JVM and I've finally tipped over the bleeding edge onto the other side where nothing works. But still, it should work, I think, so if anyone has any ideas please let me know.
Wednesday, May 14, 2008
Dragging Altio applets out of the browser
After experimenting with dragging simple applets out of the browser I tried to add the same functionality to Altio applets. This turned out to be trickier than I anticipated, mainly because AltioLive generates the APPLET tags in the HTML dynamically, setting lots of parameter values, dynamically selecting which Altio JAR and custom control JARs should be included.
I thought that I could just keep static values in the JNLP file and maintain the dynamic values in the APPLET tag, and this does work for dragging the applet out of the browser, however if you then close the browser then as far as I can tell your applet is 'magically' transformed into a Webstart application, which makes sense. This means that upon closing the browser Java will throw away your APPLET tag and rely solely on your JNLP file to support your applet, and will reinitialize your application based on that JNLP file, so if it doesn't contain all of the information necessary to your applet it will fail when Webstart takes over.
So, it looks like we are going to have to have dynamic JNLP files for AltioLive. The easiest way I can see to do this is to use JSP file that takes in a load of parameters, which are then used to fill in the JNLP and return it. We can embed a link to this JSP file, including all parameters, when we create the APPLET tag, and I have a little proof of concept of this up and running, as you can see from the screenshot below:

Unfortunately, there is another issue when closing the browser window: sessions. AltioLive relies on sessions when communicating with the server, and when the browser is closed we lose our session. I'm not sure what to do about this yet - whether we have to cope with that and re-establish a session or if we can maintain the session when switching from Applet -> Webstart.
I thought that I could just keep static values in the JNLP file and maintain the dynamic values in the APPLET tag, and this does work for dragging the applet out of the browser, however if you then close the browser then as far as I can tell your applet is 'magically' transformed into a Webstart application, which makes sense. This means that upon closing the browser Java will throw away your APPLET tag and rely solely on your JNLP file to support your applet, and will reinitialize your application based on that JNLP file, so if it doesn't contain all of the information necessary to your applet it will fail when Webstart takes over.
So, it looks like we are going to have to have dynamic JNLP files for AltioLive. The easiest way I can see to do this is to use JSP file that takes in a load of parameters, which are then used to fill in the JNLP and return it. We can embed a link to this JSP file, including all parameters, when we create the APPLET tag, and I have a little proof of concept of this up and running, as you can see from the screenshot below:

Unfortunately, there is another issue when closing the browser window: sessions. AltioLive relies on sessions when communicating with the server, and when the browser is closed we lose our session. I'm not sure what to do about this yet - whether we have to cope with that and re-establish a session or if we can maintain the session when switching from Applet -> Webstart.
Sunday, May 11, 2008
Dragging Java applets out of the browser
As we saw from the impressive JavaOne demonstrations, Sun are aiming to turn JavaFX applets into desktop widgets by allowing the user to drag them out of the browser to their desktop.
As we also saw from the number of people visiting our booth, everyone seemed to think this was a JavaFX feature. Well, it's not, and I've just got one of my JavaOne session example applets doing the same thing that was demonstrated at JavaOne, although I will admit the JavaFX demos look a damn site better than my little demo applet.
The key thing you need to accomplish dragging an applet out of a browser is the Java 6 Update 10 installed on your machine and running as the browser plugin. This means you need Firefox Beta 3 if you don't use IE. You can download the beta from http://java.sun.com/javase/downloads/index.jsp
Once you have this, then enabling applet dragging requires two things:
1 - Deploy your applet using a JNLP file (previously just used for WebStart)
2 - Add the 'draggable' parameter to your applet.
Step 1:
You can find more details on JNLP support in the new Update 10 plugin at http://java.sun.com/javase/downloads/ea/6u10/newJavaSystemProperties.jsp, but all that a JNLP file does is provide a standard mechanism for describing an applet, its dependent JAR files, its JVM parameters and JRE version requirements.
Here is the one that I am using:
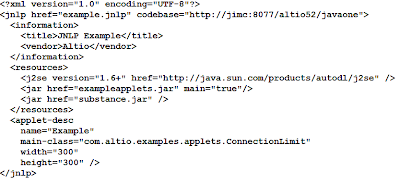
Most of this is not very interesting, and is just describing how to run the applet. What we need next is to use this JNLP file to deploy our applet, which we need to change our applet's HTML to do.
Step 2:
To deploy your applet with a JNLP file then you need to change your APPLET tag in the HTML page to reference the JNLP file, like so:
The main things to note here are the jnlp_href value, which points at our JNLP descriptor file, and the draggable parameter, which enables our new dragging functionality.
Once you have these in place, then run your applet, and when it is running try to drag it out of the browser using ALT + Left Click. If all goes well, your applet will be living large outside of the browser.
The cool thing is that the applet is not destroyed and recreated during this process - my applet can be dragged mid-connection loop to the server and the connections remain uninterrupted during the drag process, and continue once the applet is outside of the browser, as you can see below:
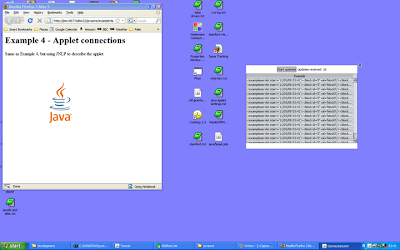
I'll try some more interesting examples soon, and the next step is to deploy AltioLive using a JNLP file and run it outside the browser, which will be very interesting.
As we also saw from the number of people visiting our booth, everyone seemed to think this was a JavaFX feature. Well, it's not, and I've just got one of my JavaOne session example applets doing the same thing that was demonstrated at JavaOne, although I will admit the JavaFX demos look a damn site better than my little demo applet.
The key thing you need to accomplish dragging an applet out of a browser is the Java 6 Update 10 installed on your machine and running as the browser plugin. This means you need Firefox Beta 3 if you don't use IE. You can download the beta from http://java.sun.com/javase/downloads/index.jsp
Once you have this, then enabling applet dragging requires two things:
1 - Deploy your applet using a JNLP file (previously just used for WebStart)
2 - Add the 'draggable' parameter to your applet.
Step 1:
You can find more details on JNLP support in the new Update 10 plugin at http://java.sun.com/javase/downloads/ea/6u10/newJavaSystemProperties.jsp, but all that a JNLP file does is provide a standard mechanism for describing an applet, its dependent JAR files, its JVM parameters and JRE version requirements.
Here is the one that I am using:
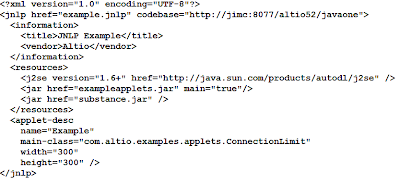
Most of this is not very interesting, and is just describing how to run the applet. What we need next is to use this JNLP file to deploy our applet, which we need to change our applet's HTML to do.
Step 2:
To deploy your applet with a JNLP file then you need to change your APPLET tag in the HTML page to reference the JNLP file, like so:
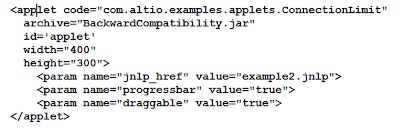
Once you have these in place, then run your applet, and when it is running try to drag it out of the browser using ALT + Left Click. If all goes well, your applet will be living large outside of the browser.
The cool thing is that the applet is not destroyed and recreated during this process - my applet can be dragged mid-connection loop to the server and the connections remain uninterrupted during the drag process, and continue once the applet is outside of the browser, as you can see below:
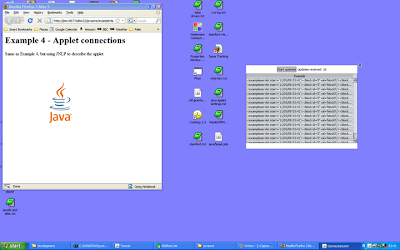
I'll try some more interesting examples soon, and the next step is to deploy AltioLive using a JNLP file and run it outside the browser, which will be very interesting.
Saturday, May 10, 2008
JavaOne, Day 4
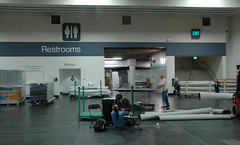
As you can see from the photo, we hung around the rapidly emptying hall until after lunch, waiting for DHL who were apparently 'going the extra mile' for us by turning up over an hour late.
I did make it to a technical session this morning though, the first one of the week, which was about designing good user interfaces. The talk was good - a bit more basic than I had hoped for though. There was a great cartoon strip representation of the differences between a customer's requirements, the engineer's ideas, the delivered product and what the customer
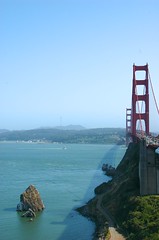
Once we were finished at JavaOne, Tom and I took a crowded bus over to the Golden Gate bridge (involving more walking than we originally planned, thanks to some rubbish unsolicited advice from a 'local'). We walked across the bridge to Marin county and back again - it's an incredible structure and it was a perfect day for walking over.
Once we'd walked back we bus + walked over to Haight-Ashbury, which does have some good points, such as a few decent bars and some great cafes, but is also incredibly tacky in places.
We finished off the day with a beer at Eddie Rickenbacker's bar on 2nd street, one of the more interesting bars I've ever been in: overhead there are 20 or so vintage motorbikes, and on the bar was one of the largest cats I've ever seen, strolling from one end to the other before settling into a basket to be spoiled and fed by staff and customers.
Labels:
javaone,
san francisco
Friday, May 09, 2008
JavaOne, Day 3

We had a visit from a customer, Tom did an impromptu Altio application for an attendee who turned up with his own data file and wanted to see it visualized using the Altio Graph control, we raffled off an Asus Eee to a lucky winner and we got rid of all the remaining Altio stress/juggling balls.
The JavaFX buzz is still the main focus of the show, and we had a few

The After Dark party later was outside, which was a bit chilly, but had Smash Mouth as the entertainment, which was pretty cool. I was a bit too shattered to really enjoy it though - it's been a long, but worthwhile week. Shame I didn't get to see any technical sessions - we underestimated the amount of effort to keep the booth ticking over - but hopefully we'll be back next year, and in greater numbers.
Thursday, May 08, 2008
Next Generation Applets
This is the video of the Sun presentation demonstrating how the new Java 6 Update 10 plugin enables applets to be dragged out of the browser to exist as desktop 'widgets'.
http://news.zdnet.com/2422-13568_22-200560.html
http://news.zdnet.com/2422-13568_22-200560.html
JavaOne, Day 2

As well as meeting an interesting set of people who visited the booth (including the lead developer on the Swing team, who knew me from a bug I'd submitted :-), Gary and I met with some people from the Java SE team that we'd dealt with before via phone/email, so it was nice to finally put faces to names.
Big news from the previous day was the demonstration of dragging a browser based JavaFX application out of the browser onto the desktop - I didn't appreciate at the time that the functionality behind that wasn't JavaFX specific - it is part of the new Java 6 update 10 functionality and is available to any applet that uses JNLP deployment descriptors, which is something I'd been playing around with for AltioLive.

That opens up an incredible number of possibilities for Altio applications: users can write AltioLive applications, deploy them via the browser and then have users drag them onto their desktops to have them available outside the browser. After years of neglecting the Java plugin, Sun have really put an incredible amount of features into the new plugin, and even if that was largely driven by JavaFX requirements, applets get the benefits of it.
Wednesday, May 07, 2008
JavaOne, Day 1

After an early pancake breakfast, Gary and I headed to the JavaOne Pavilion to do some last minute Altio booth setup and to practice our technical session presentation. At 11:30am they opened up the Pavilion to all attendees, and so we started having to deal with lots of enquiring people wanting to know who Altio was, what AltioLive does and whether they could take one or more of our free 'stress balls'.
After a couple of hours of that Gary and I did some last minute session preparation and then went to room 104 to deliver our technical session. I estimated about 80-100 attendees (it was hard to tell due to the number of lights pointed at the stage), and I thought the session went very well - everything worked, we were bang on time and people seemed interested in what we were talking about: we certainly got a lot of questions regarding applets after the session.
After our session was over we manned the Altio booth until 6:30, at which time the JavaOne Welcome session began, which meant beer and food was available for everyone, so Tom and I joined in....just to keep up appearances. Unfortunately, as I was just finished my second beer, a media crew from the Sun Developer Network website arrived at our booth and wanted a filmed, impromptu 10 minute interview about applets to put on the website. This was great news for us, but less great news for me as I was the one who had to stand in front of the camera and talk coherently and knowledgeably about AltioLive with no preparation and after a couple of beers. I think it went alright though, and apparently that visit was driven from the good response to our technical session earlier in the day, so that's great feedback.
It does seem that applets are still popular with some people, and that Sun want to re-introduce to everyone else and Altio seem to be emerging as leading advocates for using applets, which is fantastic for us and great for applets generally.
Roll on tomorrow then - just let me try and sleep off the last of my jetlag first.
Tuesday, May 06, 2008
JavaOne, Day 0
After a fraught speaker training session yesterday when I realised Sun had replaced the words 'JavaScript' and 'Java' with stock phrases, such as 'JavaScript Programming Language' in my presentation titles I had to go and get that sorted out, and then spent the afternoon doing the practice that my session coach recommended: reading each slide out loud 15 times. It was very useful, but after 4 hours doing that yesterday afternoon the combination of talking for that long and jetlag kicking in meant that I could barely think or speak and so I gave in and tried to get some sleep. As Gary and I had started the day early with an insomniac's photography walk around San Francisco I am hoping to get over the lingering jetlag today and be ready for the first full day of JavaOne tomorrow.
Monday, May 05, 2008
JavaOne, Day -1
Turns out we have 77 people registered for our Technical Session on Tuesday, which is pretty cool. We're off now to get some speaker coaching for it, so I'll post another update tomorrow.
Friday, May 02, 2008
New Altio website

We can thankfully say good riddance to the old site, which had almost no good points at all, and welcome in the new Altio site. It still has a few rough edges, and a few sections are lacking content, but it is a huge improvement, and is definitely a work in progress, not something to sit unattended for months like the old site.
www.altio.com
Sunday, April 27, 2008
Parallels

Today I tried something different and installed Ubuntu 8.04 in Parallels, and that seems to work really well too. The Parallels / OS X integration is superb with Windows (the Coherence mode allows you to seamlessly mix applications from either OS), but that is not available for Ubuntu, which is a shame, but it still runs very well. As I'm using a MacBook without a dedicated graphics card I can't seem to turn on any eye-candy for Ubuntu, but I'll see if I can get around that.
What I would like to do is take the MacBook instead of my work laptop to JavaOne, so I have every OS possible and can show Altio running in Windows, OS X and Linux just by flipping into Parallels. However, time constraints and also paranoia about having US customs decide they want to 'examine' my laptop for a few days means I probably won't be able to.
Thursday, April 24, 2008
Eating your own dogfood
I'm preparing some applet demos for my impending JavaOne technical session, and one of them is using some of the JavaOne schedule data to allow users to see live changes to session attendance, filter by session date, time, room and name.
It's a fairly simple AltioLive application, and doesn't do much more that the conference session catalog JavaOne website, which uses HTML controls and full page refreshes to search the catalog on user-specifed criteria, but it's far more interactive and user-friendly. That got me thinking: why don't Sun have applets on the JavaOne site? Certainly the usual 'barriers' to running applets on websites such as large JRE downloads for users without Java would be almost certainly be non-issues to such a pro-Java audience?
They obviously couldn't deploy anything using their current favourite rich UI solution, JavaFX, due to its bleeding-edge nature, but a 'filthy rich' Swing applet would go a long way to supporting Sun's efforts to promote Java as a viable rich internet UI tool. Much of their recent efforts rely on the new Java 6 Update 10 to fix deficiencies in applet deployment, which is only just in beta, so let's hope that when the Update 10 improvements are generally available we start seeing more applets in the wild.
Having said that, the forthcoming Altio website revamp doesn't use applets, Altio or otherwise, aside from for demo applications, and we're even planning on using Flash on the front page, so we're just as guilty as Sun.
It's a fairly simple AltioLive application, and doesn't do much more that the conference session catalog JavaOne website, which uses HTML controls and full page refreshes to search the catalog on user-specifed criteria, but it's far more interactive and user-friendly. That got me thinking: why don't Sun have applets on the JavaOne site? Certainly the usual 'barriers' to running applets on websites such as large JRE downloads for users without Java would be almost certainly be non-issues to such a pro-Java audience?
They obviously couldn't deploy anything using their current favourite rich UI solution, JavaFX, due to its bleeding-edge nature, but a 'filthy rich' Swing applet would go a long way to supporting Sun's efforts to promote Java as a viable rich internet UI tool. Much of their recent efforts rely on the new Java 6 Update 10 to fix deficiencies in applet deployment, which is only just in beta, so let's hope that when the Update 10 improvements are generally available we start seeing more applets in the wild.
Having said that, the forthcoming Altio website revamp doesn't use applets, Altio or otherwise, aside from for demo applications, and we're even planning on using Flash on the front page, so we're just as guilty as Sun.
Tuesday, April 22, 2008
Smash Mouth at JavaOne
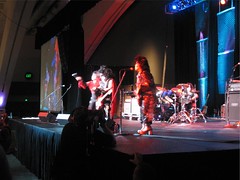
Probably not quite as amusing as last year's Mini Kiss gig, but I'm looking forward to it.
Labels:
javaone
Sunday, April 20, 2008
Google Social API demo in AltioLive

As part of our preparation for attending JavaOne next month, we've been working on some new AltioLive demos to use in our booth, and also to liven up Altio's online presence a little.
2 guys in my team spent some time working on integrating the Google Social API with AltioLive to produce a cool demo that displays the online 'identities' of any blog or other social website that you enter, and the links between them and other sites.
Try it out at http://tinyurl.com/64dkc9 either enter your own blog address, or Pownce user pages seem to work well as they mark up their links correctly.
Saturday, April 19, 2008
Blatant nepotism

My brother's band, The Gents, have a new album out (showing my age now, as I'm not sure there is a physical version, just a downloadable one). It's called 'Unzip and dial', and it's great - 'God' is my current favourite track.
Check it out: http://www.myspace.com/itsthegents or find it on iTunes
Saturday, April 12, 2008
Seeing sense
Against all the odds it seems like a UK MP has defied expectations and is showing some common. Labour MP Austin Mitchell has tabled an Early Day Motion about the harassment of people taking photographs in public places, by both private security staff and the police. Contact your MP to ask them to support Mitchell's motion (www.writetothem.com is an easy way to do it).
After the Metropolitan's ridiculous recent 'be afraid' campaign against photographers this is very welcome, and combined with the petition to Downing Street on the same subject it looks like perhaps the situation may improve soon.
After the Metropolitan's ridiculous recent 'be afraid' campaign against photographers this is very welcome, and combined with the petition to Downing Street on the same subject it looks like perhaps the situation may improve soon.
Labels:
law,
photography,
uk
Friday, April 11, 2008
Unaccustomed as I am

I am attending JavaOne again this year, although this time instead of just me there are 4 of us going, plus we're exhibiting as Altio in the JavaOne pavilion, so we can get to show people some of the cool new features we've been working on for the next release of AltioLive.
As well as all that the technical session paper I submitted has, after an initial 'alternate' status, been accepted, so Gary and I will be presenting a session entitled "Do applets have a place in Web 2.0?" on Tuesday.
If you're attending JavaOne they come and say Hi at our booth or come and listen to our talk.
Thursday, April 10, 2008
Back from the dead
Not that there's a big difference between this blog when it is active or when I have abandoned it for a year, but I've decided to return to use this instead of behaving like a small child looking at something shiny and trying other things, like Tumblr.
Subscribe to:
Posts (Atom)